[ 1, 2 ].concat([5], [7, 9]) // [ 1, 2, 5, 7, 9 ]
const new_array = old_array.concat([value1[, value2[, ...[, valueN]]]])
copyWithin()> 92%
copies part of array to another location[ 1, 2, 3, 4, 5 ].copyWithin(0,2) // [ 3, 4, 5, 4, 5 ]
arr.copyWithin(target[, start[, end]])
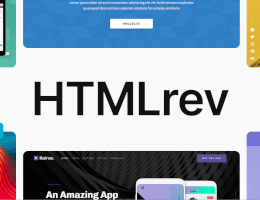
Sponsored
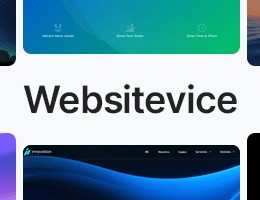
Sponsored
['a', 'b', 'c']
.entries() // Array Iterator { }
.next() // { value: (2) […], done: false }
.value // Array [ 0, "a" ]
arr.entries()
[1, 30, 40].every(val => val > 0) // true
arr.every(callback(element[, index[, array]])[, thisArg])
[1, 2, 3, 4].fill('x', 1, 3) // [ 1, "x", "x", 4 ]
arr.fill(value[, start[, end]])
[1, 10, 5, 6].filter(val => val > 5) // [ 10, 6 ]
let newArray = arr.filter(callback(element[, index, [array]])[, thisArg])
[1, 10, 5, 6].find(val => val > 5) // 10
arr.find(callback(element[, index[, array]])[, thisArg])
findIndex()> 94%
returns index of the first element, that matches test[1, 4, 5, 6].findIndex(val => val > 5) // 3
arr.findIndex(callback( element[, index[, array]] )[, thisArg])
[1, [2, [3, [4]]]].flat(2) // [ 1, 2, 3, [4] ]
const new_array = arr.flat([depth]);
[[2], [4], [6], [8]].flatMap(val => val/2) // [ 1, 2, 3, 4 ]
var new_array = arr.flatMap(function callback(currentValue[, index[, array]]) {
// return element for new_array
}[, thisArg])
[ 1, 2, 3 ].forEach(val => console.log(val)) // 1 // 2 // 3
arr.forEach(callback(currentValue [, index [, array]])[, thisArg])
includes()> 95%
determines if array includes a certain value[ 1, 2, 3 ].includes(3) // true
arr.includes(valueToFind[, fromIndex])
[ 1, 2, 3 ].indexOf(3) // 2
arr.indexOf(searchElement[, fromIndex])
[ "x", "y", "z" ].join(" - ") // "x - y - z"
arr.join([separator])
['a', 'b', 'c']
.keys() // Array Iterator { }
.next() // { value: 0, done: false }
.value // 0
arr.keys()
lastIndexOf()> 95%
returns last index at which given element can be found[ 1, 2, 3, 1, 0].lastIndexOf(1) // 3
arr.lastIndexOf(searchElement[, fromIndex])
[ 2, 3, 4 ].map(val => val * 2) // [ 4, 6, 8 ]
let new_array = arr.map(function callback( currentValue[, index[, array]]) {
// return element for new_array
}[, thisArg])
const arr = [ 1, 2, 3 ]
arr.pop() // returns: 3 // arr is [ 1, 2 ]
arr.pop()
const arr = [ 1, 2, 3 ]
arr.push(1) // returns: 4 // arr is [ 1, 2, 3, 4 ]
arr.push(element1[, ...[, elementN]])
[ 'a', 'b', 'c' ].reduce((acc, curr) => acc + curr, 'd') // "dabc"
arr.reduce(callback( accumulator, currentValue[, index[, array]] )[, initialValue])
reduceRight()> 95%
executes a reducer function from right to left, resulting in single output value[ 'a', 'b', 'c' ].reduceRight((acc, curr) => acc + curr, 'd') // "dcba"
arr.reduceRight(callback(accumulator, currentValue[, index[, array]])[, initialValue])
[ 1, 2, 3 ].reverse() // [ 3, 2, 1 ]
arr.reverse()
const arr = [ 1, 2, 3 ]
arr.shift() // returns: 1 // arr is [ 2, 3 ]
arr.shift()
[ 1, 2, 3, 4 ].slice(1, 3) // [ 2, 3 ]
arr.slice([begin[, end]])
[ 1, 2, 3, 4 ].some(val => val > 3) // true
arr.some(callback(element[, index[, array]])[, thisArg])
[ 1, 2, 3, 4 ].sort((a, b) => b - a) // [ 4, 3, 2, 1 ]
arr.sort([compareFunction])
const arr = [ 1, 2, 3, 4 ]
arr.splice(1, 2, 'a') // returns [ 2, 3 ] // arr is [ 1, "a", 4 ]
let arrDeletedItems = array.splice(start[, deleteCount[, item1[, item2[, ...]]]])
toLocaleString()> 95%
elements are converted to Strings using toLocaleString and are separated by locale-specific String (eg. “,”)[1.1, 'a', new Date()].toLocaleString('EN') // "1.1,a,5/18/2020, 7:58:57 AM"
arr.toLocaleString([locales[, options]]);
toString()> 95%
returns a string representing the specified array[ 'a', 2, 3 ].toString() // "a,2,3"
arr.toString()
const arr = [ 1, 2, 3 ]
arr.unshift(0, 99) // returns 5 // arr is [ 0, 99, 1, 2, 3 ]
arr.unshift(element1[, ...[, elementN]])
['a', 'b', 'c']
.values() // Array Iterator { }
.next() // { value: "a", done: false }
.value // "a"
arr.values()